HTML5 Local Storage
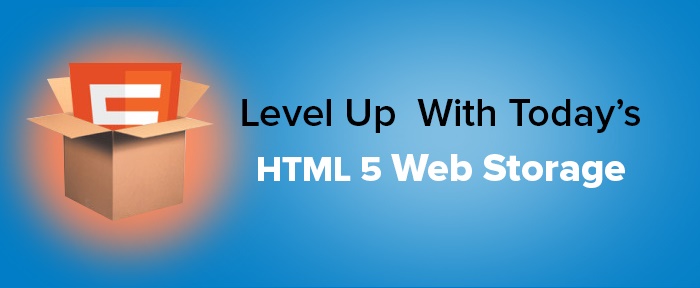

by admin
The Local Storage mechanism is a method through which string key/value pairs can be securely stored and later retrieved for use. The goal of this addition is to provide a comprehensive way through which interactive applications can be built (including advanced abilities, such as being able to work “offline” for extended periods of time).
Reasons to choose Local Storage : –
- Data accessed over the internet can never be as fast as accessing data locally.
- Data accessed over internet is not secure.
- HTML5 storage is on client.
Persistent Local Storage :-
- Native client applications use operating system to store data such as preferences or runtime state.
- Stored in registry, INI files, XML or other places using key/value pairs.
- Web applications can’t do this.
What are Cookies? :-
- Invented early in Web history as a way to store persistent data (“magic cookies”).
- Small pieces of information about a user stored by Web server as text files on user’s computer.
- Can be temporary or persistent.
- Included with every HTTP request – slows down the application by transmitting same information repeatedly.
- Sends unencrypted data over internet with every HTTP request.
- Limited to 4KB data Example: filling out a text form field.
Cookies not enough due to following reasons:-
- Require more storage space.
- On the client.
- Beyond page refresh.
- Not transmitted to server.
HTML5 Storage :-
- Provides standardized API.
- Implemented natively.
- Consistent in all the browsers.
- HTML5 storage is also named as “Web Storage”.
- Previously part of HTML5 specifications.
- Split into its own specification.
- Different browsers may call it “Local Storage” or “DOM Storage”.
There are two types of storages which are used in different situations:
- Session Storage
- Local Storage
Session Storage: Session storage is designed for the situation where the user is making a single transaction but the other transactions are also carried out in the separate windows.
Example: The user has visited a site to buy plane tickets and has opened the two different windows of the same site. If the site used cookies to keep the track of the users action to buy the ticket, and if the user navigate to both the pages time and again. In this case the ticket purchased by the user will be leaked from one window to the other, and the user will buy two tickets for the same flight unknowingly.
HTML5 introduces the sessionStorage attribute which would be used by the sites to add data to the session storage which can be accessed by any page from the same site opened in that window i.e. session and as soon as you close the window, session would be lost.
Following is the code which would set a session variable and access that variable:
<!DOCTYPE HTML>
<html>
<body>
<script type="text/javascript">
if( sessionStorage.hits ){
sessionStorage.hits = Number(sessionStorage.hits) +1;
}else{
sessionStorage.hits = 1;
}
document.write("Total Hits :" + sessionStorage.hits );
</script>
<p>Refresh the page to increase number of hits.</p>
<p>Close the window and open it again and check the result.</p>
</body>
</html>
What is Local Storage?: The Local Storage is designed for storage that spans multiple windows, and lasts beyond the current session. In particular, Web applications may wish to store megabytes of user data, such as entire user-authored documents or a user’s mailbox, on the client side for performance reasons. Again, cookies do not handle this case well, because they are transmitted with every request.
Example: HTML5 has introduced the localStorage attribute which will be used to access a page’s local storage area with no time limit and this local storage will be available to you whenever you would use that page again.
Following is the code which would set a local storage variable and access that variable every time this page is accessed, even next time when you open the window:
<!DOCTYPE HTML>
<html>
<body>
<script type="text/javascript">
if( localStorage.hits ){
localStorage.hits = Number(localStorage.hits) +1;
}
else{
localStorage.hits = 1;
}
document.write("Total Hits :" + localStorage.hits );
</script>
<p>Refresh the page to increase number of hits.</p>
<p>Close the window and open it again and check the result.</p>
</body>
</html>
Here is the list of lowest versions of different web browsers that support HTML5 Web Storage :-
IE 8+, Firefox 3.5+, Safari 4.0+, Chrome 4.0+, Opera 10.5+, IPhone 2.0+, Android 2.0+
Access through localStorage object on global window object. Before using, detect whether browser supports it.
Check for HTML5 Storage :-
function supports_html5_storage() {
try {
return 'localStorage' in window
&& window['localStorage'] !== null;
} catch (e) {
return false;
}
}
Or we can also use Modernizr plugin:-
if (Modernizr.localstorage) {
// window.localStorage is available!
} else {
// no native support for HTML5 storage :(
// maybe try dojox.storage or a third-party solution
}
Localstorage object :-
setItem( )
getItem( )
removeItem( )
clear( )
Limitation :- Most of the limitations on local storage are similar to cookie limitations, and can be controlled by the user, such as:
- According to the current HTML 5 Storage specification browsers must set a limit of 5MB per origin (host or domain).
- Blocking third-party storage — only allowing storage from the originating domain.
- Deleting storage contents — browsers should allow customers to delete storage data just like they can cookie data.
- Black and whitelists of origin data — Browsers may have the ability to put the domains on a blacklist and never store data from the whitelist to allow storage in an unrestrained manner.
Recommended Posts
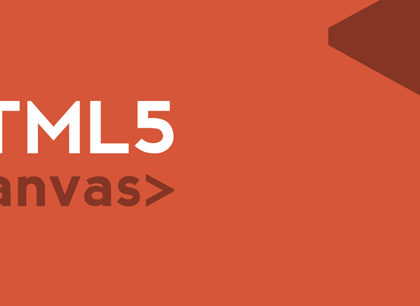
HTML5 Canvas
August 13, 2015
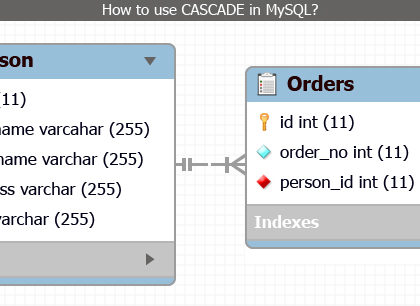
How to use Cascade in MySQL
August 13, 2015
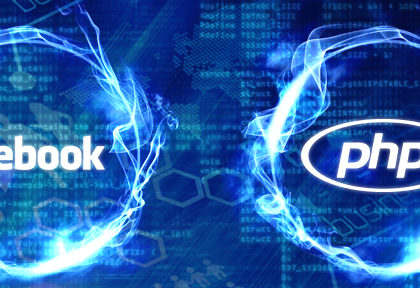
Facebook Connect in Php
August 13, 2015